Fieldset for Sitemagic CMS
The example below demonstrates how fieldsets can be used to logically seperate different sections of functionality within the same extension using the
SMFieldset class.
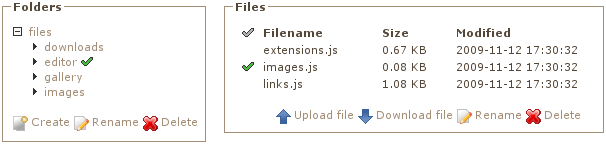
A fieldset also serves another purpose - it allows the ENTER key to be bound to a specific action given by a link button. So when ENTER is pressed in e.g. a contact form, the Fieldset triggers a click on the associated Send button, rather than just performing a normal post back, which doesn't instruct Sitemagic CMS to do anything.
The following example creates a fieldset with 3 input fields. When ENTER is pressed in one of the input fields, the Create button is triggered.
// Create input fields used to enter account information
$txtUsername = new SMInput("UniqueIdUsername", SMInputType::$Text);
$txtPass1 = new SMInput("UniqueIdPass1", SMInputType::$Password);
$txtPass2 = new SMInput("UniqueIdPass2", SMInputType::$Password);
// Create button used to create new account
$buttonCreate = new SMLinkButton("UniqueIdCreate");
$buttonCreate->SetTitle("Create account");
// Construct sign-up form as HTML
$html = "";
$html .= "<br>Username: " . $txtUsername->Render();
$html .= "<br>Choose password: " . $txtPass1->Render();
$html .= "<br>Password again: " . $txtPass2->Render();
$html .= "<br>" . $buttonCreate->Render();
// Create fieldset, set content (sign-up form), set the legend title,
// and have the Create button triggered when ENTER is pressed
// in one of the input fields.
$fieldset = new SMFieldset("UniqueIdFieldset");
$fieldset->SetContent($html);
$fieldset->SetLegend("Create new account");
$fieldset->SetPostBackControl($buttonCreate->GetClientId());
// Button is triggered when it is clicked, or when ENTER is pressed
if ($buttonCreate->PerformedPostBack() === true)
{
// Create account here..
}